Billing Request (Instant Bank Pay feature)
Take a one-off Instant bank payment
Goal - Create an Instant Bank Pay using Billing Request
Engineering complexity - Easy
Time taken - 15 minutes
Note 🇬🇧 🇩🇪 🇫🇷 🇮🇪 Instant Bank Pay supports GBP and EUR, available for transactions in GBP with your customers in the UK, and available for EUR with your customers in Germany, France, and Ireland. For payments in the UK, we also support Direct Funds Settlement.
Create a Billing Request that specifies the payment you want to take from your customer using the payment_request
object.
Our example will take a payment from an existing customer, by specifying the customer ID under links.customer
. Leave this blank to set up a totally new customer.
Use the Create a Billing Request endpoint:
1$client = new \GoCardlessPro\Client(array(
2 'access_token' => 'your_access_token_here',
3 'environment' => \GoCardlessPro\Environment::SANDBOX
4));
5
6$client->billingRequests()->create([
7 "params" => [
8 "payment_request" => [
9 "description" => "First Payment",
10 "amount" => "500",
11 "currency" => "GBP",
12 "app_fee" => "500",
13 ],
14 ]
15]);
You’ll receive a full Billing Request resource back.
It will look like this:
{
"billing_requests": {
"id": "BRQ123",
"status": "pending",
"mandate_request": null,
"payment_request": {
"currency": "GBP",
"amount": "500",
"description": "£5 Top Up",
"scheme": "faster_payments"
},
"links": {
"customer": "CU00016VR36GVW",
"customer_billing_detail": "CBD000010M15PAC",
"organisation": "OR123"
}
}
}
The Billing Request is currently pending, meaning we need to complete the outstanding actions before it can be fulfilled. We can use Billing Request Flows to generate a checkout flow that guides the payer through these actions.
Integrators building custom payment pages should read the Billing Request actions to understand what actions are available, and how to complete them.
If you complete actions yourself, you can resume this guide to have Billing Request Flows complete whatever remains.
Goal - Create a Billing Request Flow that can be used for your customer to authorise payments
Engineering complexity - Easy
Time taken - 15 minutes
Billing Request Flows can be created against Billing Requests, and provide an entry into a hosted GoCardless flow that completes whatever actions remain against the request.
Create a Billing Request Flow to retrieve a link that can be provided to your customer to complete the request:
1$client = new \GoCardlessPro\Client(array(
2 'access_token' => 'your_access_token_here',
3 'environment' => \GoCardlessPro\Environment::SANDBOX
4));
5
6$client->billingRequestFlows()->create([
7 "params" => [
8 "redirect_uri" => "https://my-company.com/landing",
9 "exit_uri" => "https://my-company.com/exit",
10 "links" => [
11 "billing_request" => "BRQ000010NMDMH2"
12 ]
13 ]
14]);
This returns a new flow, which has an authorisation_url
you should send to your customer:
1{
2 "billing_request_flows": {
3 "authorisation_url": "https://pay.gocardless.com/billing/static/flow?id=<brf_id>",
4 "lock_customer_details": false,
5 "lock_bank_account": false,
6 "auto_fulfil": true,
7 "created_at": "2021-03-30T16:23:10.679Z",
8 "expires_at": "2021-04-06T16:23:10.679Z",
9 "redirect_uri": "https://my-company.com/completed",
10 "links": {
11 "billing_request": "BRQ123"
12 }
13 }
14}
15
Share your authorisation link from the response in Step 02, via a button on your website, SMS, email, or any other way you like.
Preview what your customer will see by following the steps below.
Goal - Retain your customers with an Exit URI
Engineering complexity - Easy
Time taken - 5 minutes
Some customers may not be able to authorise the open banking transaction through the Billing Request Flow. This may happen if they are unable to use their mobile, they aren’t set up with online banking or the bank’s open banking API may be down.
To retain these customers and let them pay another way, you may pass an Exit URI which will send them wherever you choose in order to complete the transaction. It’s as simple as providing the address you’d like us to return the customer to.
Provide an Exit URI when creating the Billing Request Flow
You may pass a exit_uri
when creating the Billing Request Flow, the same way you pass the redirect_uri
.
Note: We recommend that you return customers to your checkout page, at the point of choosing a payment method.
1$client = new \GoCardlessPro\Client(array(
2 'access_token' => 'your_access_token_here',
3 'environment' => \GoCardlessPro\Environment::SANDBOX
4));
5
6$client->billingRequestFlows()->create([
7 "params" => [
8 "redirect_uri" => "https://my-company.com/landing",
9 "exit_uri" => "https://my-company.com/exit",
10 "links" => [
11 "billing_request" => "BRQ000010NMDMH2"
12 ]
13 ]
14]);
This returns a new flow which includes the exit_uri being passed:
1{
2 "billing_request_flows": {
3 "authorisation_url": "https://pay.gocardless.com/billing/static/flow?id=<brf_id>",
4 "lock_customer_details": false,
5 "lock_bank_account": false,
6 "auto_fulfil": true,
7 "created_at": "2021-03-30T16:23:10.679Z",
8 "expires_at": "2021-04-06T16:23:10.679Z",
9 "redirect_uri": "https://my-company.com/completed",
10 "links": {
11 "billing_request": "BRQ123"
12 }
13 }
14}
15
Customers will be sent to this URI when they click on the Exit URI in the Billing Request Flow.
Customer uses the Exit URL to return to your checkout
The Exit URI is used in two places:
Instant Bank Pay for British customers
Instant Bank Pay for German customers
Instant Bank Pay in the UK
The Exit URL is shown if a customer is unable to find their bank, when searching the institution selector.
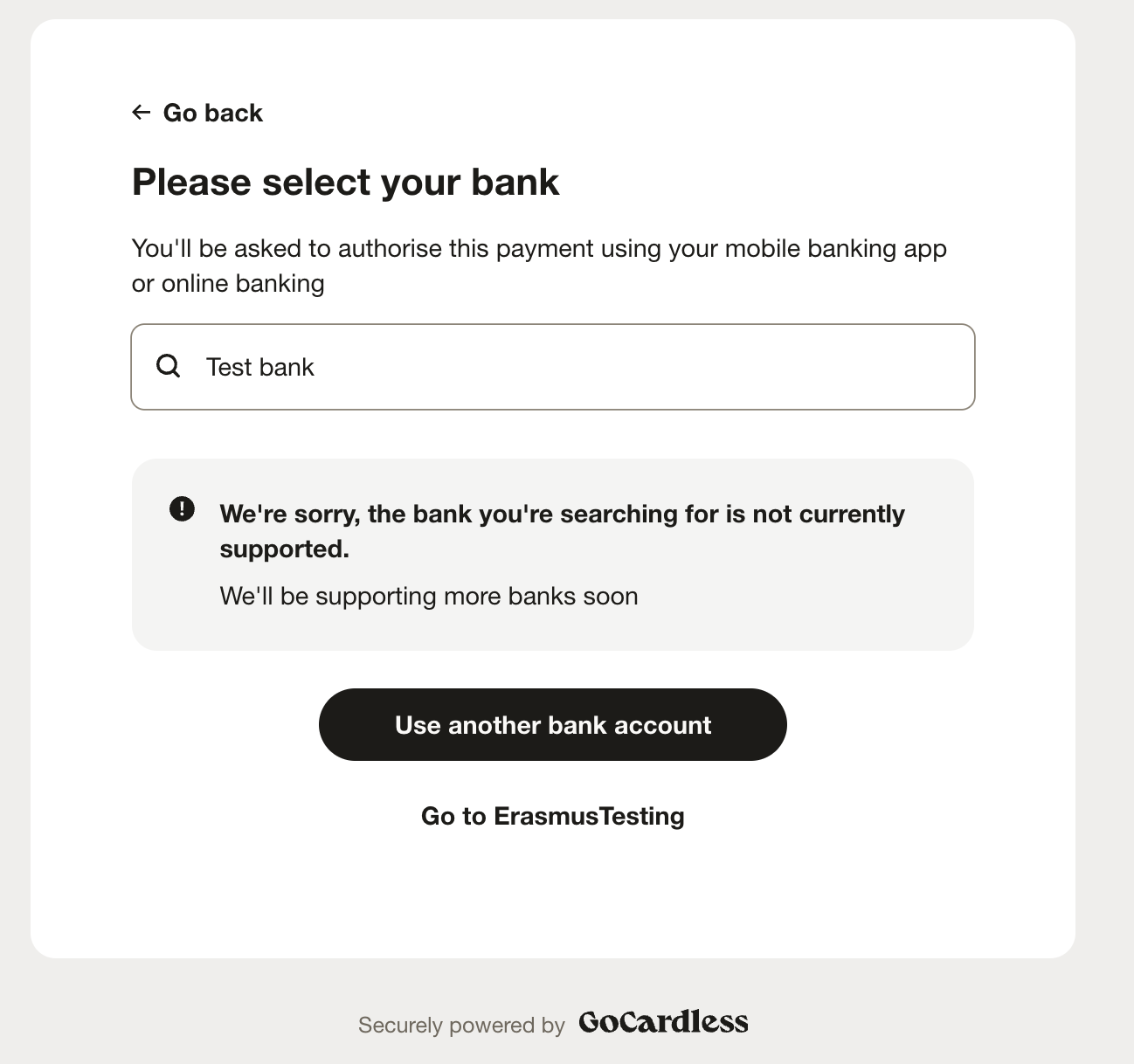
Instant Bank Pay in Germany
The Exit URL is shown if a customer provides an IBAN which isn’t supported.
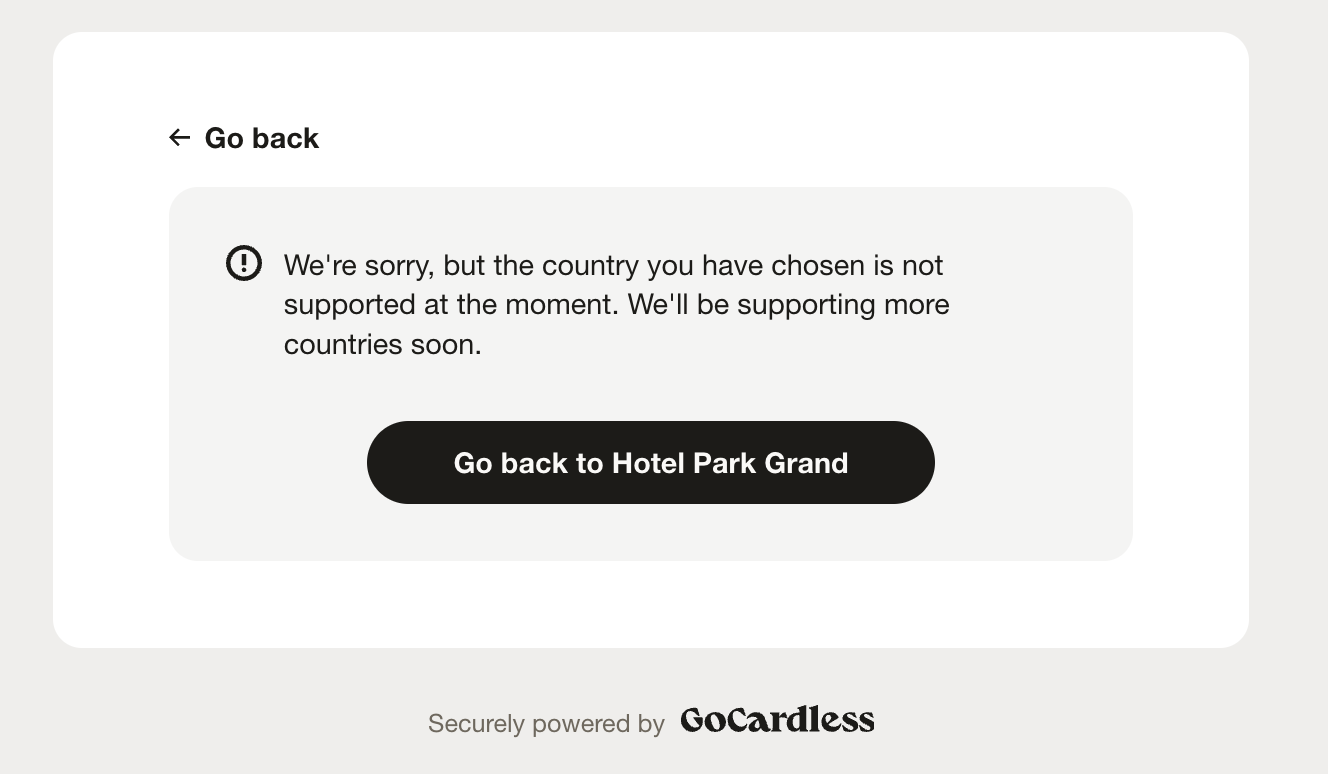
As mentioned before if certain actions are already completed, or can be completed by another required action then we can skip them. The most common cases where a component may be skipped in the flow are as follows:
A Customer already exists- in this case, we do not show the CollectCustomerDetails page however the user may be allowed to edit these details unless the customer has been locked (see locking components below)
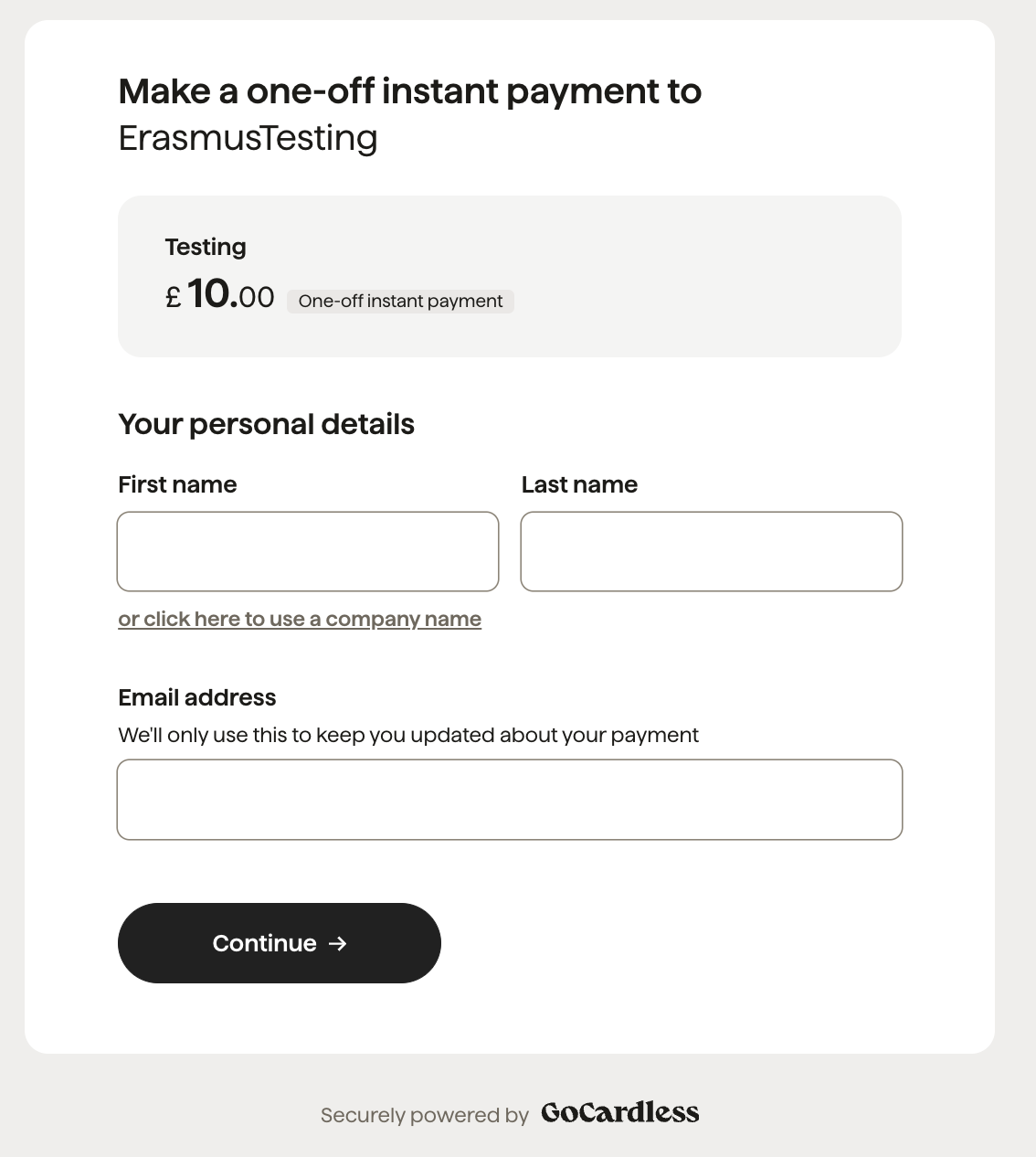
A Bank account already exists- in this case, we do not show the CollectBankAccount page however the user may be allowed to create a new bank account and use that for the payment unless the bank account has been locked (see locking components)
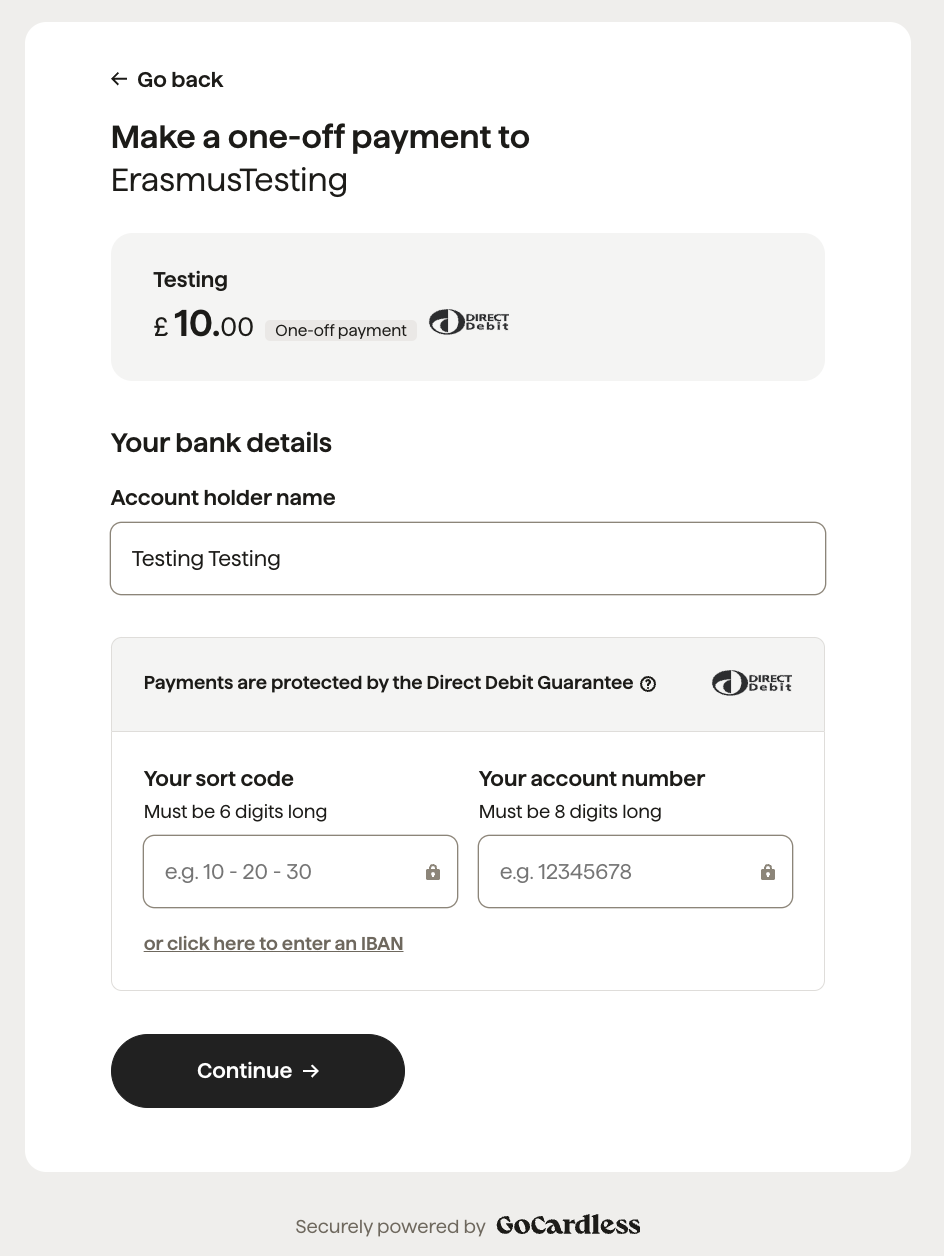
Bank authorisation allows the collection of banks- in this case, if there are no existing banks, we will not ask the user to pick the bank account, instead, we use the details returned from the stage to create it
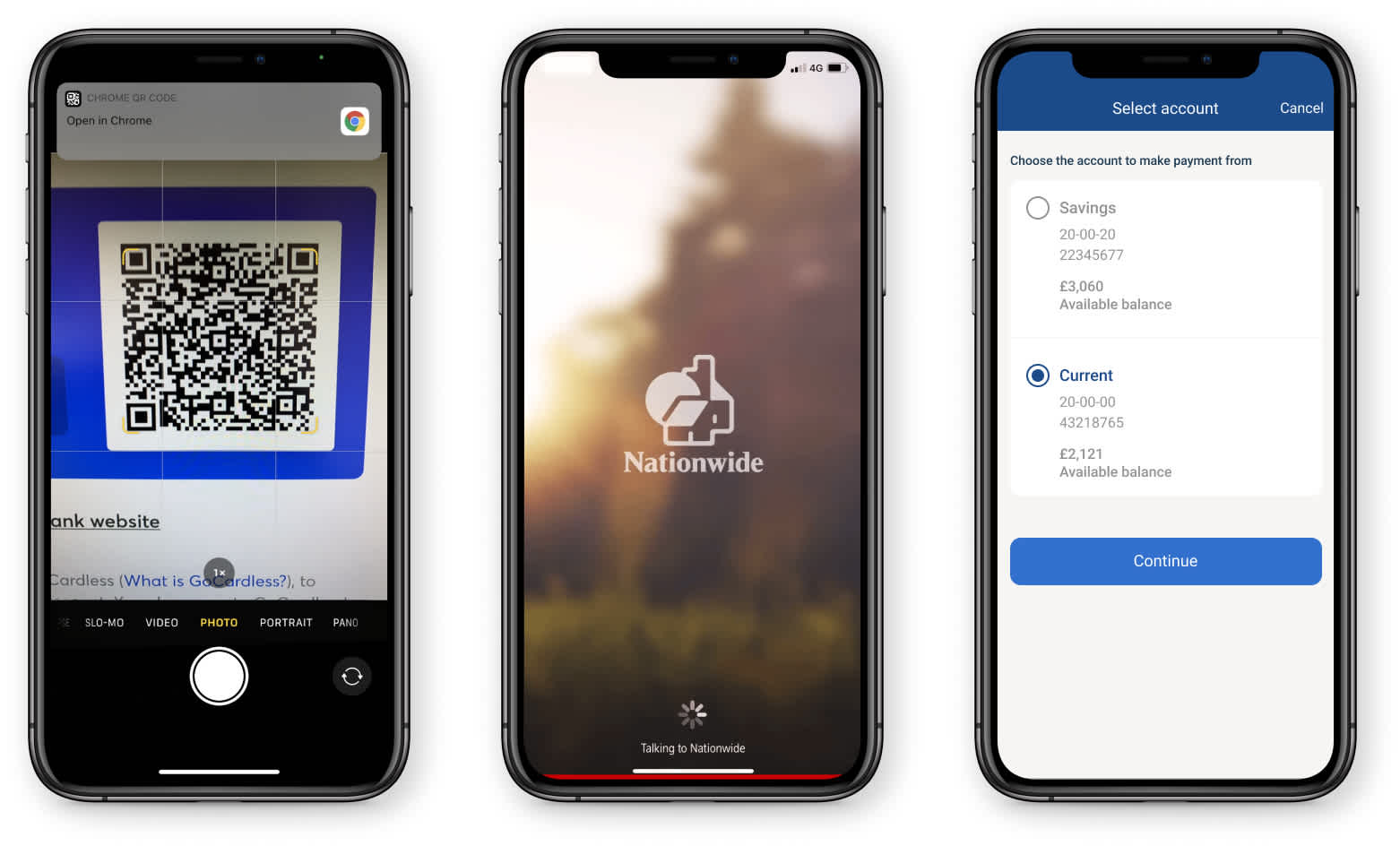
Existing customers or banks can be attached to the Billing Request when it is created, an example of this might be when creating a “top-up” single payment for a customer you already have a relationship with. See API reference
Along with knowing the customer's details and the bank account you may wish to “lock” the request to only allow the customer to use those details. This allows you to ensure the payment is taken using details you have already collected for this customer in your own systems.
You can lock the customer, the bank account or both. This is done when creating the Billing Request Flow by setting lock_customer_details
or lock_bank_account
to true.
If a linked resource is locked the following happens:
Customer locked - The CollectCustomerDetails page is not shown and the customer cannot open them for editing
Bank account locked - The CollectBankAccount page is not shown and the customer cannot add another bank account to use instead
If both are locked then we skip almost every page and go directly to the confirmation page within the BankHandoff component. All the payer needs to do is confirm and possibly authenticate with the bank if it is required.
For mandate only requests, you may also "lock" the currency and scheme that the customer pays in. By default, mandate only requests have the currency/scheme "unlocked" so that the customer can set up their mandate in any of your available schemes. If you lock the currency, however, they will only be able to pay with the scheme you specified when creating the Billing Request. This can be useful if you only want to allow a customer to pay in one specific scheme.
This can be achieved by setting lock_currency
to true on the Billing Request Flow. In this case a currency selector will not show in the payment page UI for the payer, and they will be unable to change currency.
What the customer will see
Collect customer details in order to complete the billing request.
Note: this screen is skipped if the details already exist, or the customer details have been locked.
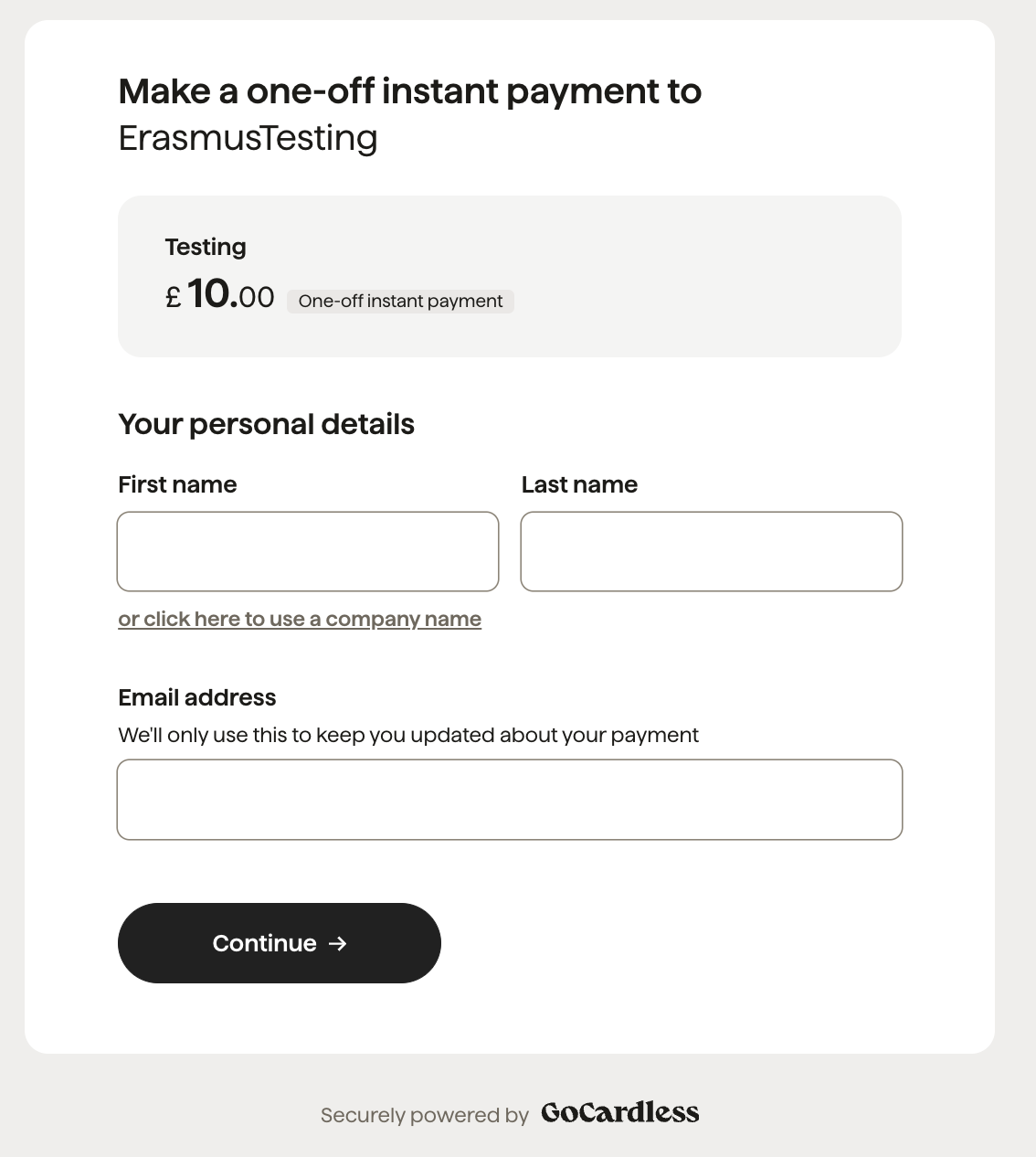
Select the Bank account account in order for GC to take a payment.
Note: this screen is skipped if the details already exist or the bank account is locked.
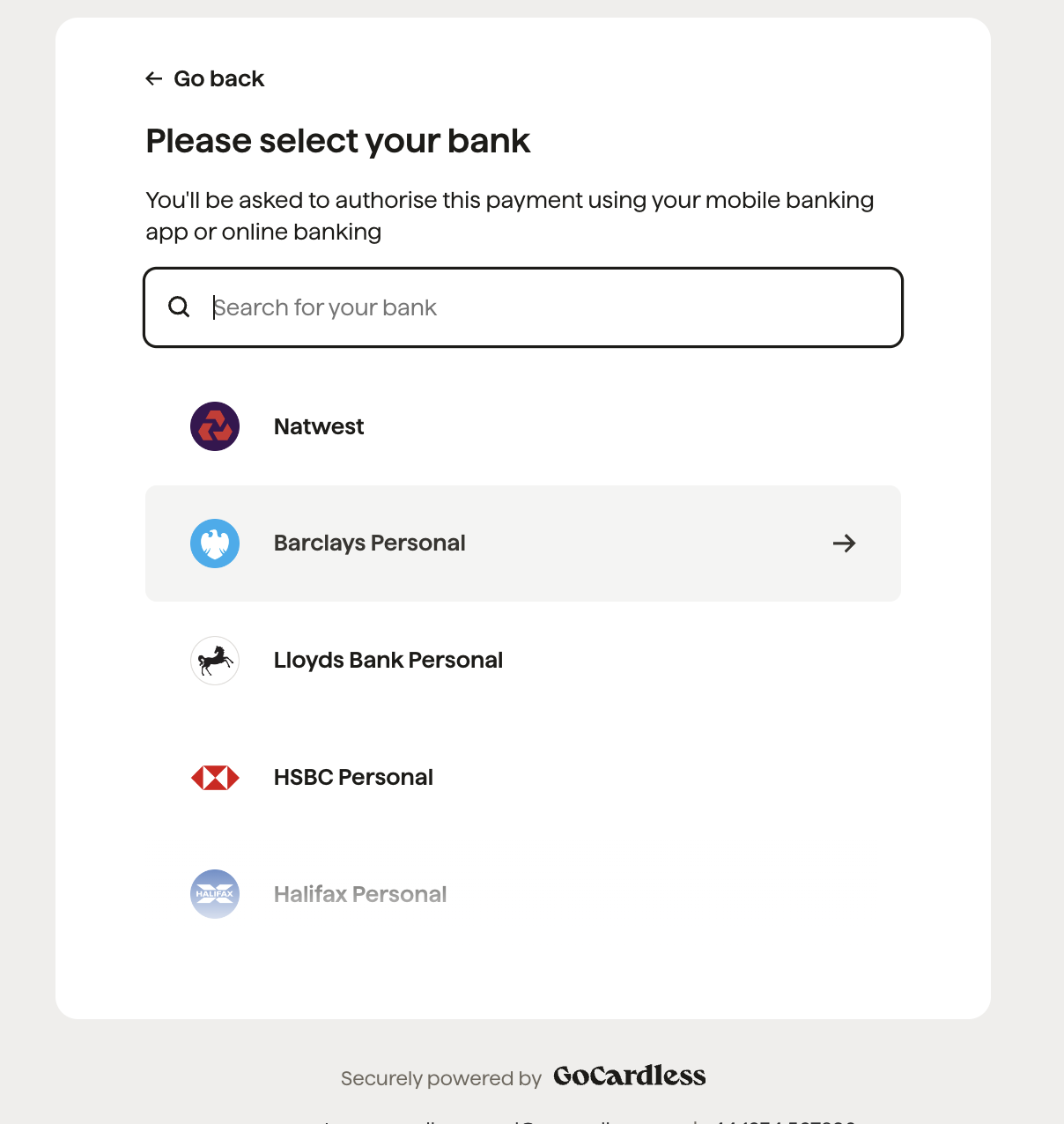
Preview the payment and bank, before authorising anything.
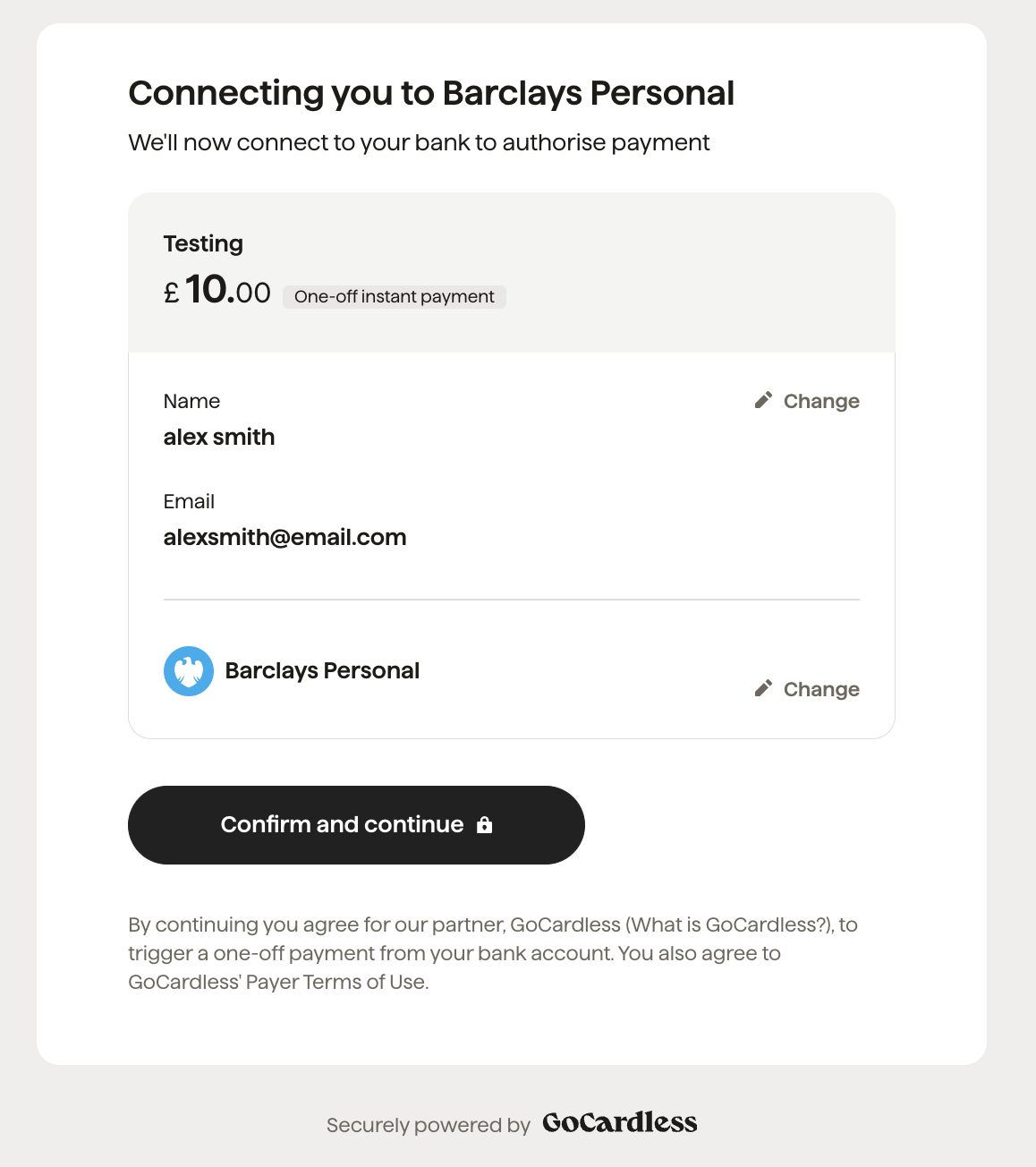
Go to their bank to complete the authorisation. Customers can use the QR code to jump directly into their mobile banking app (this is the best UX in most cases) or opt to continue on their desktop.
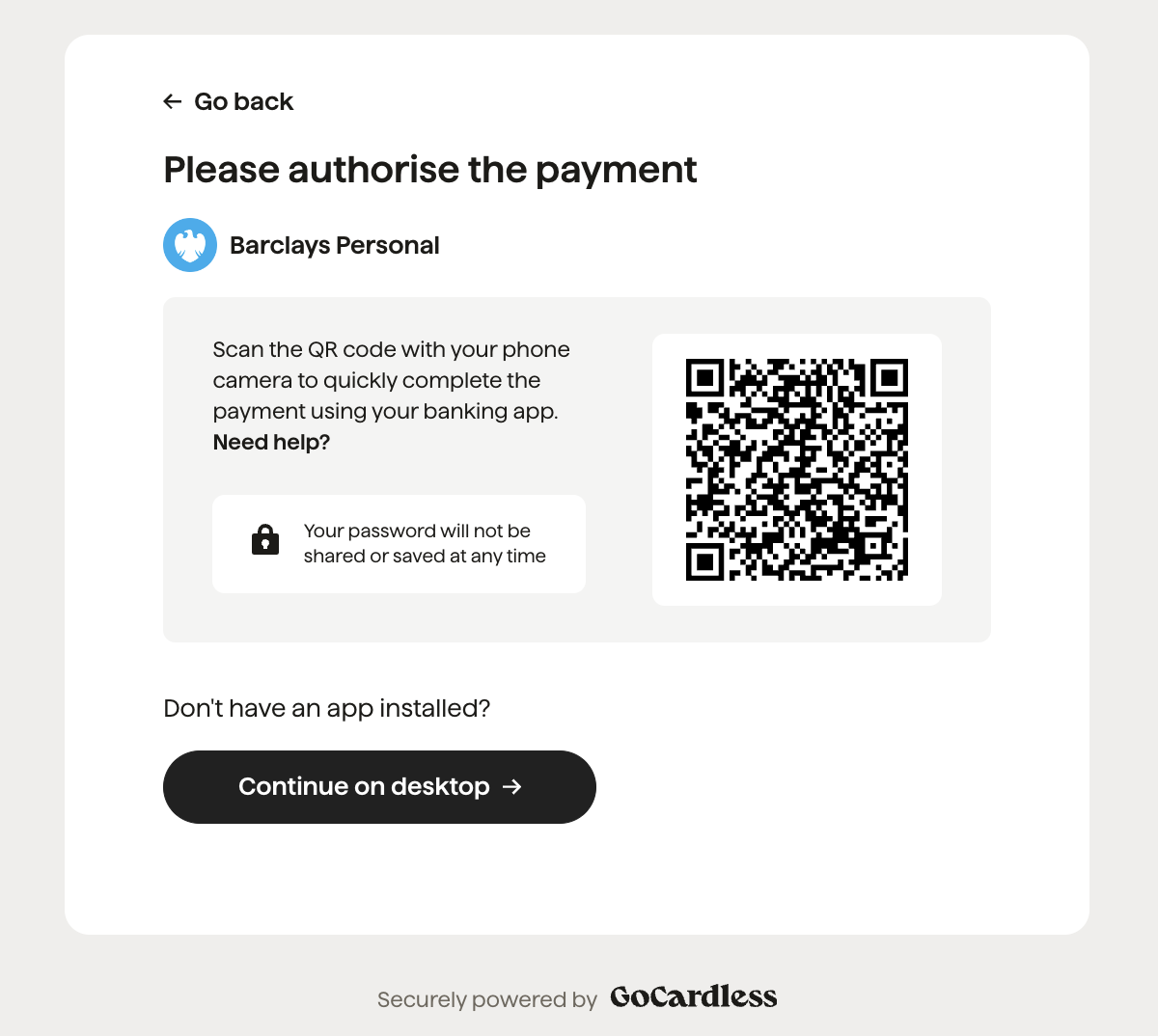
View confirmation that the payment has been successful once the bank authorisation is complete.
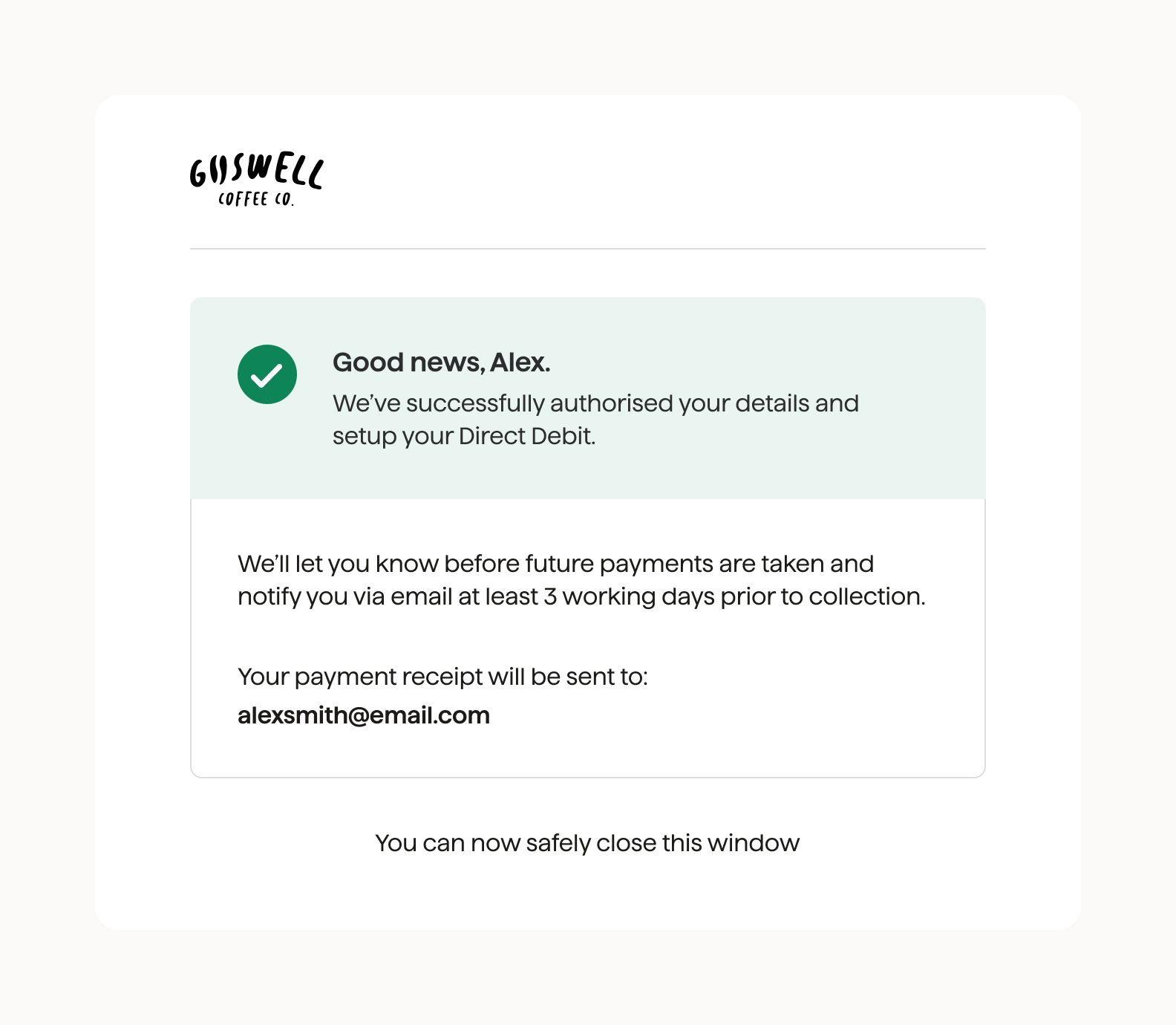
Done!
The payment has now been completed, and the Billing Request is fulfilled
. Because this is an instant bank payment, the funds are confirmed within minutes of the request going through and leave the customer’s bank account immediately.
What’s next?
First instant payment with mandate Direct Debit set up
Get started
For partners
Go to Partner PortalTo learn more about technical and UX requirements